This is a two-series article which discusses some simple strategies to generate Random data for your REST Services using some simple tools available from the Linux command line. Also, we will show some minimal tool you can install for a wider variety of choices.
Meaningful Random values for REST
Before delving into the tools available for generating random values in Linux, it’s essential to set clear expectations regarding the nature and purpose of these tools. Randomly generated values lack inherent meaning or significance. While this is suitable for scenarios requiring unpredictability, it also means the random data may not resemble real-world scenarios accurately.
On the other hand, Random value generators offer a simple and accessible solution, requiring no installation of complex tools on your machine. This makes them well-suited for simple use cases where a quick and straightforward solution is needed.
That being said, let’s dive into the generation of random text, numbers or attributes for your REST Services! In the first article of this series we will use common Linux tools to generate random text or numbers. Also, we will show how to use a Cloud Service to provide a more sophisticated set of Data for our REST Services.
The RANDOM function
Purpose: generate a random integer value
Firstly, we will mention the RANDOM unix function. The RANDOM
variable in Linux is a built-in variable in Bash that generates a random integer between 0 and 32767 each time it is referenced. It’s often used for generating random numbers in scripts or command-line operations.
If you want to define an upper bound for the RANDOM value, simply use us it as follows:
# Generate a random number between 0 and 99 random_number=$((RANDOM % 100))
For example, here is how to call a REST Service passing in the JSON Payload a Random attribute:
# Generate a random number between 0 and 99 random_number=$((RANDOM % 100)) # Create a JSON payload with the random number json_payload="{\"attribute\": $random_number}" # Send the POST request to a hypothetical REST endpoint curl -X POST \ -H "Content-Type: application/json" \ -d "$json_payload" \ http://example.com/api/resource
If you want to explore more combination of curl for your REST Services then we recommend checking this article: REST Services cURL cheatsheet
The uuidgen command
Purpose: generate a random alphanumeric string
This command generates a random UUID (Version 4 UUID) and prints it to the standard output. A UUID is a 128-bit number represented as a 36-character string, usually separated by hyphens into five groups (8-4-4-4-12 characters).
For example:
uuidgen | tr -d '-' 5de64b76c27a47f88e0eb43a10c584be
Please note that we are piping the output with tr
command . This command with the -d
option removes the hyphens from the UUID result. Here is, for example, how to use it to set an Header attribute in a REST Service:
UUID=$(uuidgen | tr -d '-') curl -X POST https://api.example.com/orders \ -d '{"product_id": 1234, "quantity": 5}' \ -H 'Content-Type: application/json' \ -H "X-UUID: $UUID"
Generate a string from /dev/random
Purpose: generate a random alphanumeric string
By reading from /dev/urandom
and formatting it hexadecimal format you can generate actual random string attributes. For example:
hexdump -n 10 -v -e '/1 "%02X"' -e '/10 "\n"' /dev/urandom 7323EC4F20AF5E750489
In this example, we requested a 10 bytes random string with -n 10
option. Since each byte is represented by two hexadecimal characters, it translates into a 20 characters long random string.
For example, you can use it as follows in a REST POST command:
# Capture the output of hexdump in the USERNAME variable USERNAME=$(hexdump -n 5 -v -e '/1 "%02X"' -e '/5 "\n"' /dev/urandom) # Use the USERNAME variable in a curl POST request curl -X POST \ -H "Content-Type: application/json" \ -d '{"username": "'"$USERNAME"'"}' \ http://example.com/api/resource
Use a Password generator tool
Purpose: generate a random alphanumeric string
There are several password generators tool available for Linux. One which is pretty common is pwmake
. This tool is a simple utility which includes only one option: the amount of entropy bits used to generate the password.
$ pwmake 128 BUd&Yz-Yh4fAdcYzew#3GByzgEh
Here is the command in action:
# Generate a password using pwmake password=$(pwmake 128) # Perform a curl POST request with the generated password as an attribute curl -X POST -H "Content-Type: application/json" -d "{\"password\":\"$password\"}" http://your-api-endpoint
Use https://randomuser.me/api/ Service
Purpose: generate a complete User random structure in JSON format
If you have internet access, then you can try the https://randomuser.me/api/ public
API which generates a full username structure with lots of data in JSON format. You generate is as follows:
curl https://randomuser.me/api/
This service provides a wealth of attributes, not just name and surname and I invite you to have a look at it. Here’s just a partial overview of the JSON result that this API can produce:
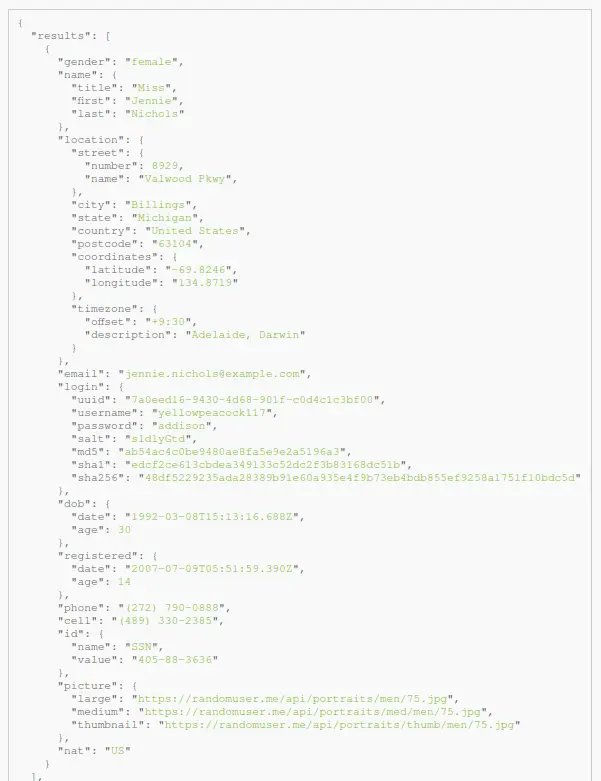
Personally, I’ve found quite useful to add it as a function in my bash_rc file and export the random attributes in the current shell. For example:
random_user_info() { local api_response api_response=$(curl -s https://randomuser.me/api/) export FIRST_NAME=$(echo "$api_response" | jq -r '.results[0].name.first') export LAST_NAME=$(echo "$api_response" | jq -r '.results[0].name.last') export EMAIL=$(echo "$api_response" | jq -r '.results[0].email') export USERNAME=$(echo "$api_response" | jq -r '.results[0].login.username') export PASSWORD=$(echo "$api_response" | jq -r '.results[0].login.password') export PHONE=$(echo "$api_response" | jq -r '.results[0].phone') }
Then, we will show how to use it to make a POST request to the kitchensink WildFly demo:
$ random_user_info $ curl -d '{"name":"$FIRST_NAME","email":"$EMAIL","phoneNumber":"$PHONE"}' -H "Content-Type: application/json" -X POST http://localhost:8080/kitchensink/rest/members
Besides curl, you can find this API incredibly powerful if you use it in combination with jQuery’s $.ajax() function to make an AJAX request:
$.ajax({ url: 'https://randomuser.me/api/', dataType: 'json', success: function(data) { console.log(data); } });
Conclusion
This article was a walk through the generation of random text,numbers from the Linux Command line and how to use it to provide data attributes to REST Services. In the next article of this series we will show how to code a JBang script to provide a wider set of attributes that you use as source of data for your Services. Stay tuned!
Found the article helpful? if so please follow us on Socials